2025-03-03 Web Development
Optimized CustomImage Component for Next.js
By O. Wolfson
When working with images in a Next.js project, using the built-in next/image
component is the recommended approach for optimized loading, automatic resizing, and improved performance. However, customizing next/image
to fit various layouts while maintaining responsiveness and loading enhancements can be tricky.
This article introduces a reusable CustomImage
component (custom-image.tsx
) designed to provide seamless aspect ratio maintenance, a skeleton loader, and caption support.
MDX Compatibility
The CustomImage
component is fully compatible with MDX content, making it easy to embed optimized images directly within Markdown files. This is particularly useful for blog posts, documentation, and other content-driven projects in Next.js.
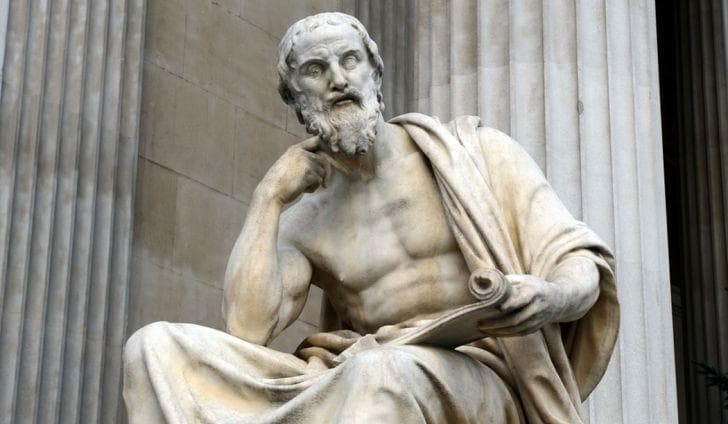
Example Usage in MDX
When using CustomImage
in an MDX file, simply import the component and embed it within the Markdown content:
This approach allows you to seamlessly integrate images within your content while leveraging Next.js optimizations.
Component Overview
The CustomImage
component, implemented in custom-image.tsx
, wraps Next.js's next/image
component and enhances it with additional features:
- Maintains Aspect Ratio: Dynamically calculates the aspect ratio from
width
andheight
props, ensuring the image scales correctly. - Skeleton Loader: Displays a subtle loading animation until the image fully loads.
- Automatic Responsive Behavior: Uses absolute positioning to maintain layout integrity.
- Optional Caption: Displays a descriptive text below the image if provided.
Code Implementation
Key Features and Benefits
1. Preserves Aspect Ratio
By wrapping the NextImage
component in a div
with a dynamic padding-bottom
based on the aspect ratio, the image maintains its expected proportions without layout shifts.
2. Skeleton Loader for Better UX
A subtle loading animation is displayed while the image is fetching, creating a smoother user experience.
3. Improved Performance
By leveraging next/image
, the component benefits from Next.js’s automatic optimizations, such as lazy loading and efficient image rendering.
4. Optional Caption Support
Adding a caption below the image makes it more descriptive and useful for accessibility and SEO.
Naming Considerations
Naming the component CustomImage
inside custom-image.tsx
avoids confusion with the built-in next/image
import. This change improves clarity and maintains best practices for naming conventions in Next.js projects.
Conclusion
This reusable CustomImage
component enhances the standard next/image
with additional usability features, ensuring responsive, optimized, and aesthetically pleasing image rendering in a Next.js project. By implementing a skeleton loader, maintaining aspect ratios, and supporting captions, this component improves user experience while leveraging Next.js’s powerful image optimization capabilities.